Key Takeaways
1. REST APIs leverage HTTP's full capabilities for scalable web communication
REST tells us how the Web achieves its great scale.
Architectural constraints enable scalability. REST (Representational State Transfer) is the architectural style of the World Wide Web, designed to enable massive scalability. It achieves this through key constraints:
- Client-server separation of concerns
- Stateless interactions
- Uniform interface (including resource identification, manipulation through representations, self-descriptive messages, and hypermedia controls)
- Layered system allowing intermediaries
- Caching to reduce latency
- Optional code-on-demand for client extensibility
These constraints work together to create a distributed system capable of handling enormous numbers of interactions while remaining flexible and performant.
2. URIs should uniquely identify resources without revealing implementation details
Every character within a URI counts toward a resource's unique identity.
Design clear, hierarchical URIs. URIs (Uniform Resource Identifiers) are the cornerstone of REST APIs, uniquely identifying each resource. Best practices for URI design include:
- Use forward slashes to indicate hierarchical relationships
- Prefer lowercase letters
- Use hyphens to improve readability
- Avoid file extensions
- Use plural nouns for collections
- Use singular nouns for specific resources
- Employ consistent naming conventions across the API
Well-designed URIs should be intuitive and self-describing, allowing developers to understand the resource structure without needing extensive documentation. However, clients should treat URIs as opaque identifiers and not attempt to parse meaning from them directly.
3. HTTP methods define standard interactions with resources
A REST API must not compromise its design by misusing HTTP's request methods in an effort to accommodate clients with limited HTTP vocabulary.
Use HTTP methods consistently. The standard HTTP methods provide a uniform interface for interacting with resources:
- GET: Retrieve a resource representation
- POST: Create a new resource in a collection or execute a controller
- PUT: Update an existing resource or create a new resource in a store
- DELETE: Remove a resource
- HEAD: Retrieve metadata about a resource
- OPTIONS: Discover available interactions for a resource
Each method has specific semantics and expected behavior. For example, GET should be safe and idempotent, while POST may have side effects. Consistently applying these methods across your API design enhances predictability and interoperability.
4. Metadata in HTTP headers enhances API functionality and performance
Caching is one of the most useful features built on top of HTTP.
Leverage HTTP headers for metadata. HTTP headers provide a wealth of functionality for REST APIs:
- Content-Type: Specifies the media type of the request or response body
- ETag: Enables efficient caching and conditional requests
- Cache-Control: Directs caching behavior for improved performance
- Authorization: Supports various authentication schemes
- Accept: Allows content negotiation for different representations
Proper use of headers can significantly improve API performance, security, and flexibility. For example, effective use of caching headers can reduce server load and improve response times, while authentication headers enable secure access control.
5. Resource representations should use consistent formats and hypermedia controls
REST API clients should be encouraged to rely on the self-descriptive features of a REST API.
Design self-describing representations. Resource representations should be consistent and self-describing:
- Use standard formats like JSON or XML
- Include hypermedia controls (links) to guide clients through available actions
- Employ consistent structures for common elements like errors
- Use descriptive field names and appropriate data types
- Include metadata about the representation (e.g., schema information)
Hypermedia controls are particularly important, as they allow the API to guide clients through the application state. This enables looser coupling between clients and servers, improving the API's evolvability.
6. Versioning, security, and composition strategies improve API flexibility
OAuth is an HTTP-based authorization protocol that enables the protection of resources.
Design for evolution and security. Key strategies for flexible and secure APIs include:
- Versioning: Use schema versioning rather than URI versioning
- Security: Implement OAuth or API management solutions for access control
- Partial responses: Allow clients to request only needed fields
- Embedded resources: Enable clients to retrieve related resources in a single request
- Error handling: Provide consistent, informative error responses
These strategies allow APIs to evolve over time without breaking existing clients, while also providing the security and efficiency features required by modern applications.
7. JavaScript clients require special considerations for cross-origin requests
CORS is an alternative to JSONP that supports all request methods.
Enable cross-origin access. Browser security restrictions can pose challenges for JavaScript clients accessing APIs from different domains. Two main approaches address this:
- JSONP (JSON with Padding): Allows read-only access by exploiting script tag behavior
- CORS (Cross-Origin Resource Sharing): Provides full read/write access with browser support
CORS is the more robust and standardized approach, supporting all HTTP methods. By implementing CORS, APIs can securely allow access from JavaScript applications hosted on different domains, enabling rich web applications and mashups.
Last updated:
FAQ
What's "REST API Design Rulebook" by Mark Masse about?
- REST API Design Guide: The book serves as a style guide and reference for designing REST APIs, providing a set of rules to help developers create consistent and effective APIs.
- REST Fundamentals: It explains the Representational State Transfer (REST) architectural style, which is the foundation of how the Web operates, focusing on scalability and resource modeling.
- Practical Application: The book offers practical advice on implementing REST principles in API design, covering topics like URI design, HTTP methods, and media types.
Why should I read "REST API Design Rulebook"?
- Comprehensive Resource: It provides a thorough understanding of REST API design principles, making it a valuable resource for both beginners and experienced developers.
- Practical Insights: The book offers actionable rules and examples that can be directly applied to real-world API design projects.
- Industry Relevance: Understanding REST API design is crucial for modern web development, and this book equips readers with the knowledge to create scalable and maintainable APIs.
What are the key takeaways of "REST API Design Rulebook"?
- REST Principles: The book emphasizes the importance of adhering to REST principles like statelessness, client-server architecture, and uniform interfaces.
- Design Consistency: It highlights the need for consistent URI design, proper use of HTTP methods, and clear media type definitions to ensure API usability and scalability.
- Hypermedia as Engine: The concept of Hypermedia as the Engine of Application State (HATEOAS) is crucial for enabling dynamic interactions within REST APIs.
What are the best quotes from "REST API Design Rulebook" and what do they mean?
- "REST tells us how the Web achieves its great scale." This quote underscores the significance of REST in enabling the scalability of the Web, which is a core theme of the book.
- "A REST API is a type of web server that enables a client to access resources that model a system’s data and functions." It highlights the role of REST APIs in providing structured access to web resources.
- "Designing a REST API can sometimes feel more like an art than a science." This reflects the nuanced nature of API design, where creativity and adherence to principles must be balanced.
How does Mark Masse define REST in "REST API Design Rulebook"?
- Architectural Style: REST is described as an architectural style that defines a set of constraints for building scalable web services.
- Key Constraints: These include client-server architecture, statelessness, cacheability, and a uniform interface.
- Resource-Centric: REST focuses on resources, which are identified by URIs and manipulated through representations.
What is the significance of URIs in REST API design according to Mark Masse?
- Resource Identification: URIs are crucial for uniquely identifying resources within a REST API, forming the backbone of the resource model.
- Design Rules: The book provides specific rules for URI design, such as using forward slashes for hierarchy and avoiding file extensions.
- Opacity Principle: URIs should be treated as opaque identifiers by clients, ensuring flexibility in API evolution.
How does "REST API Design Rulebook" address HTTP methods?
- Method Semantics: The book explains the specific semantics of HTTP methods like GET, POST, PUT, and DELETE in the context of REST APIs.
- Proper Usage: It emphasizes the importance of using HTTP methods correctly to maintain the transparency and predictability of API interactions.
- Avoiding Tunneling: The book advises against misusing methods like GET and POST to tunnel other request methods, which can undermine protocol transparency.
What role do media types play in REST API design as per Mark Masse?
- Content Description: Media types describe the format of data within HTTP messages, guiding clients on how to process the content.
- Application-Specific Types: The book advocates for using application-specific media types to convey the semantics of resource representations.
- Negotiation Support: It encourages supporting media type negotiation to allow clients to request preferred formats and schemas.
How does Mark Masse suggest handling versioning in REST APIs?
- New Concepts, New URIs: New URIs should be introduced only for new concepts, not for versioning existing resources.
- Schema Management: Versioning of representational forms should be managed through schema documents, allowing backward compatibility.
- Entity Tags: ETags are recommended for managing representational state versions, providing a fine-grained versioning mechanism.
What security measures does "REST API Design Rulebook" recommend for REST APIs?
- OAuth Protocol: The book suggests using OAuth for secure authorization, allowing users to share resources without exposing credentials.
- API Management Solutions: It discusses the use of API management solutions and reverse proxies to protect resources and manage cross-cutting concerns.
- Resource Protection: Emphasizes the importance of securing sensitive resources, especially those associated with specific clients or users.
How does "REST API Design Rulebook" address client concerns?
- Partial Responses: The book recommends using URI query components to support partial responses, allowing clients to request only relevant data.
- Embedded Resources: It suggests enabling clients to embed linked resources within responses, facilitating efficient data retrieval.
- Hypermedia Processing: Provides guidance on processing hypermedia links and relations to enable dynamic client interactions.
What are the final thoughts of Mark Masse in "REST API Design Rulebook"?
- State of the Art: The book acknowledges the current limitations of REST API development tools and frameworks, advocating for more standardized approaches.
- Uniform Implementation: It proposes a configuration-driven engine for REST API implementation, reducing the need for boilerplate code.
- Future Vision: Envisions a future where REST APIs are designed using graphical tools, enhancing developer productivity and consistency.
Review Summary
REST API Design Rulebook receives mixed reviews. Many readers find the first half valuable for REST API design principles, but criticize the second half's focus on WRML, the author's proposed framework. Positive aspects include clear rules for API design, especially in early chapters. Criticisms include the book's brevity, repetitiveness, and overemphasis on WRML. Some readers suggest using it as a reference rather than reading cover-to-cover. Overall, the book is seen as a good introduction to REST API design, but potentially outdated and overly opinionated in parts.
Similar Books
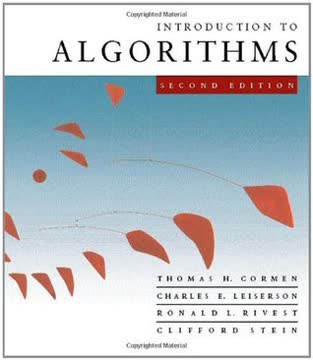
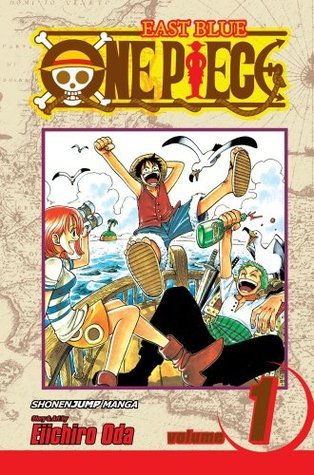
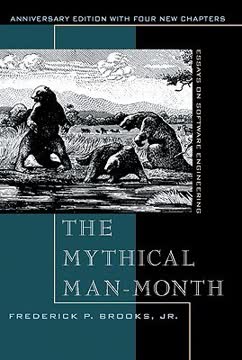
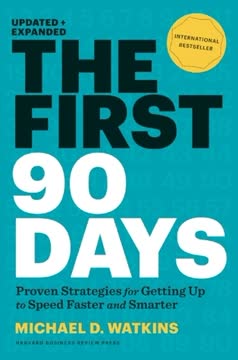
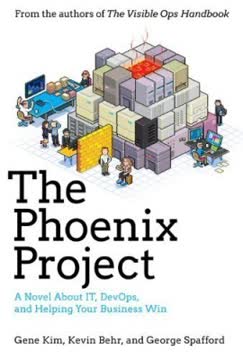
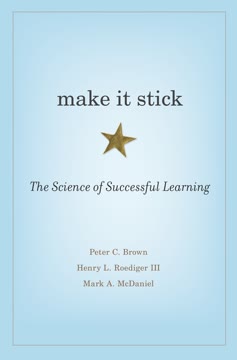
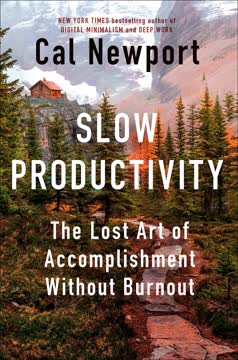
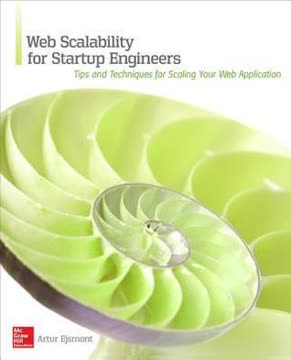
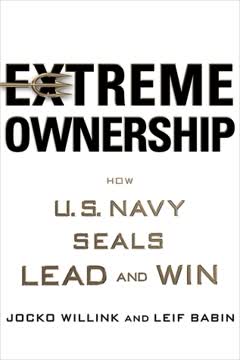
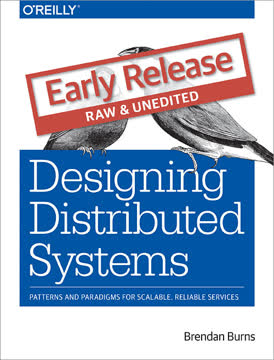
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.