Key Takeaways
1. Coding Interviews are About Problem-Solving, Not Just Coding
At most of the top tech companies (and many other companies), algorithm and coding problems form the largest component of the interview process. Think of these as problem-solving questions.
Beyond Syntax. Coding interviews aren't merely about writing syntactically correct code; they're about demonstrating your ability to dissect a problem, devise an efficient algorithm, and articulate your thought process. Interviewers want to see how you approach unfamiliar challenges and whether you can adapt your knowledge to new situations.
Problem-solving skills are paramount. The ability to break down complex problems into smaller, manageable parts is crucial. This involves identifying the core requirements, considering edge cases, and exploring different solution strategies. It's not just about getting the right answer; it's about showing how you think.
Communication is key. Talking through your thought process, explaining your reasoning, and asking clarifying questions are all essential components of a successful interview. Interviewers want to understand how you arrive at your solutions and how well you collaborate.
2. Analytical Skills: The Core of Interview Success
Analytical skills: Did you need much help solving the problem? How optimal was your solution? How long did it take you to arrive at a solution? If you had to design/architect a new solution, did you structure the problem well and think through the tradeoffs of different decisions?
Efficiency and optimality. Interviewers assess your ability to develop efficient algorithms, considering both time and space complexity. An optimal solution demonstrates a deep understanding of data structures and algorithms.
Trade-off analysis. Often, there are multiple ways to solve a problem, each with its own set of trade-offs. Interviewers want to see that you can weigh these trade-offs and make informed decisions based on the specific requirements of the problem. For example:
- Choosing between a faster algorithm that uses more memory and a slower algorithm that uses less memory
- Selecting a data structure that offers quick lookups but slower insertions
Structured approach. A well-structured approach to problem-solving involves breaking down the problem into smaller, manageable parts, identifying the core requirements, and considering edge cases. This demonstrates a systematic and organized thought process.
3. Coding Skills: Translate Algorithms into Clean Code
Were you able to successfully translate your algorithm to reasonable code? Was it clean and well-organized? Did you think about potential errors? Did you use good style?
Code clarity and organization. Interviewers evaluate the readability and maintainability of your code. Clean, well-organized code is easier to understand, debug, and modify.
Error handling and edge cases. Thinking about potential errors and handling them gracefully is a sign of a careful and thorough developer. This includes considering edge cases and boundary conditions. For example:
- Handling null or empty inputs
- Preventing integer overflows
- Validating user input
Coding style and conventions. Adhering to established coding conventions and using good style demonstrates professionalism and attention to detail. This includes using meaningful variable names, writing clear comments, and following consistent indentation.
4. Technical Knowledge: Computer Science Fundamentals are Key
Do you have a strong foundation in computer science and the relevant technologies?
Data structures and algorithms. A solid understanding of fundamental data structures and algorithms is essential for solving coding interview problems. This includes:
- Arrays and linked lists
- Trees and graphs
- Sorting and searching algorithms
- Hash tables
Big O notation. Being able to analyze the time and space complexity of algorithms using Big O notation is crucial for evaluating their efficiency. This allows you to compare different solutions and choose the most optimal one.
Relevant technologies. Depending on the role and company, you may also be expected to have knowledge of specific technologies, such as databases, web frameworks, or cloud computing platforms.
5. Experience Matters: Showcase Your Technical Decisions
Have you made good technical decisions in the past? Have you built interesting, challenging projects? Have you shown drive, initiative, and other important factors?
Project complexity and impact. Interviewers want to see that you've worked on projects that are technically challenging and have had a meaningful impact. This demonstrates your ability to tackle real-world problems.
Technical decision-making. Be prepared to discuss the technical decisions you've made in the past, explaining the reasoning behind your choices and the trade-offs involved. This shows that you can think critically and make informed decisions.
Initiative and drive. Interviewers are looking for candidates who are proactive, self-motivated, and eager to learn. This can be demonstrated through personal projects, open-source contributions, or other activities outside of work or school.
6. Culture Fit and Communication: Be Personable and Clear
Do your personality and values fit with the company and team? Did you communicate well with your interviewer?
Personality and values. Companies want to hire people who will be a good fit for their culture and values. This means being respectful, collaborative, and open to feedback.
Communication skills. Being able to communicate your ideas clearly and concisely is essential for working effectively in a team. This includes being able to explain complex technical concepts in a way that is easy to understand.
Active listening. Pay attention to what your interviewer is saying and ask clarifying questions to ensure that you understand the problem. This shows that you are engaged and interested in the conversation.
7. Mastering Big O: Essential for Algorithm Efficiency
Big O time is the language and metric we use to describe the efficiency of algorithms. Not understanding it thoroughly can really hurt you in developing an algorithm.
Understanding asymptotic runtime. Big O notation describes how the runtime of an algorithm scales as the input size grows. It's crucial to understand the common Big O times, such as O(1), O(log N), O(N), O(N log N), and O(N^2).
Dropping constants and non-dominant terms. When analyzing the runtime of an algorithm, you should drop the constants and non-dominant terms. For example, O(2N) is simplified to O(N), and O(N^2 + N) is simplified to O(N^2).
Space complexity. Big O notation can also be used to describe the amount of memory or space required by an algorithm. Understanding space complexity is just as important as understanding time complexity.
8. Optimize with BUD: Bottlenecks, Unnecessary Work, Duplicated Work
To crack the coding interview, you need to prepare with real interview questions. You must practice on real problems and learn their patterns. It's about developing a fresh algorithm, not memorizing existing problems.
Bottleneck identification. Pinpointing the part of your algorithm that is slowing down the overall runtime is crucial for optimization. Focus your efforts on improving the efficiency of this bottleneck.
Eliminating unnecessary work. Look for steps in your algorithm that are not essential for solving the problem. Removing these steps can significantly improve the runtime.
Avoiding duplicated work. Identify computations that are being performed multiple times and find ways to cache or reuse the results. This can be particularly effective in recursive algorithms.
9. The Interview is Relative: Performance Against Other Candidates
Receiving an offer is not about solving questions flawlessly (very few candidates do!). Rather, it is about answering questions better than other candidates.
Relative assessment. Interviewers evaluate your performance relative to other candidates on the same question. It's not about getting a perfect score; it's about demonstrating that you are a stronger problem-solver than your peers.
Embrace challenging questions. Getting a hard question isn't necessarily a bad thing. When it's harder for you, it's harder for everyone else, too. Focus on demonstrating your problem-solving skills, even if you don't arrive at a perfect solution.
Don't stress over imperfections. It's okay to not be flawless. Interviewers understand that everyone makes mistakes. What matters is how you handle those mistakes and whether you can learn from them.
10. Behavioral Questions: Specificity and Structure are Key
The focus of Cracking the Coding Interview is algorithm, coding, and design questions. Why? Because while you can and will be asked behavioral questions, the answers will be as varied as your resume.
Specificity over generalities. When answering behavioral questions, provide specific examples from your past experiences. This makes your answers more credible and allows the interviewer to assess your skills and accomplishments.
Structured responses. Use frameworks like the S.A.R. (Situation, Action, Result) method to structure your answers. This helps you organize your thoughts and communicate your experiences effectively.
Focus on your role. Remember that interviews are an individual assessment. Focus on your specific contributions and impact, rather than just describing what your team accomplished.
11. Prepare for Acquisitions: Interview Like Your Company Depends On It
These interviews can carry enormous importance. They have three different roles:
- They can make or break acquisitions. They are often the reason a company does not get acquired.
- They determine which employees receive offers to join the acquirer.
- They can affect the acquisition price (in part as a consequence of the number of employees who join).
Acquisition interviews matter. If your startup is being acquired, the technical interviews are not just a formality. They can significantly impact the acquisition price and whether you receive an offer to join the acquiring company.
Team preparation is essential. Prepare as a team, studying individually, in pairs, and through mock interviews. Focus on core computer science concepts and practice challenging algorithm questions.
Don't wait until the last minute. Acquisition interviews often come up suddenly. Start preparing your team well in advance to maximize your chances of success.
Last updated:
Review Summary
Cracking the Coding Interview receives mostly positive reviews, with readers praising its effectiveness in preparing for technical interviews and landing jobs at top tech companies. Many find it comprehensive and valuable for refreshing CS concepts. Some criticisms include its focus on Java, advanced difficulty, and potential for making interviews formulaic. Readers recommend supplementing with other resources and warn that working through the entire book can be time-consuming. Overall, it's considered a must-read for those serious about tech careers, especially early in their journey.
Similar Books
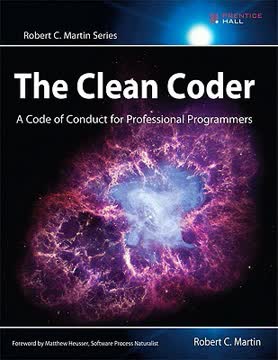
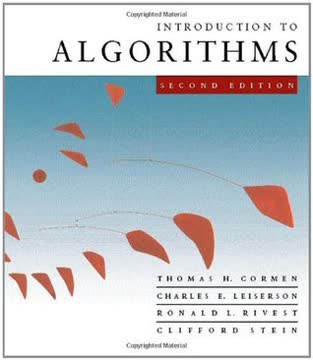
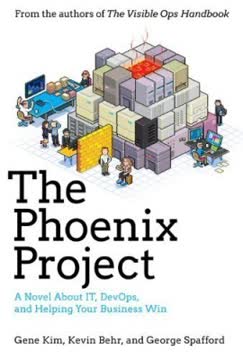
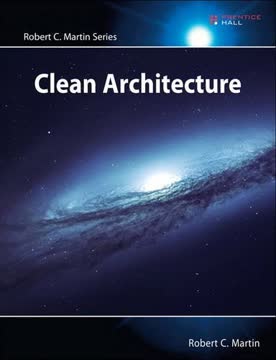
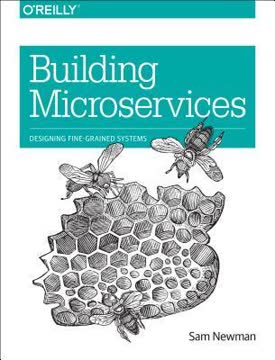
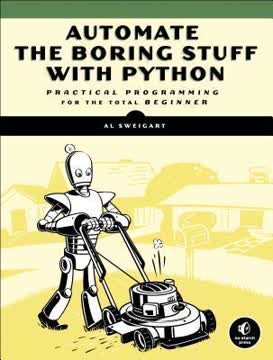
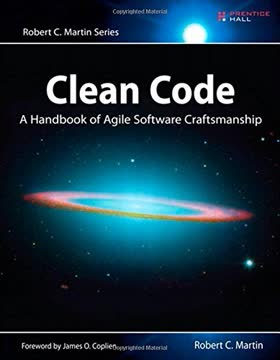
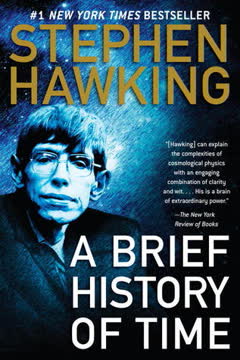
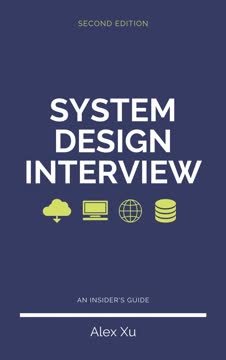
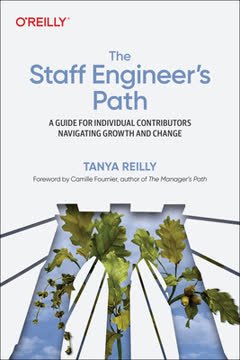
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.